An uncommon case and why you may need it
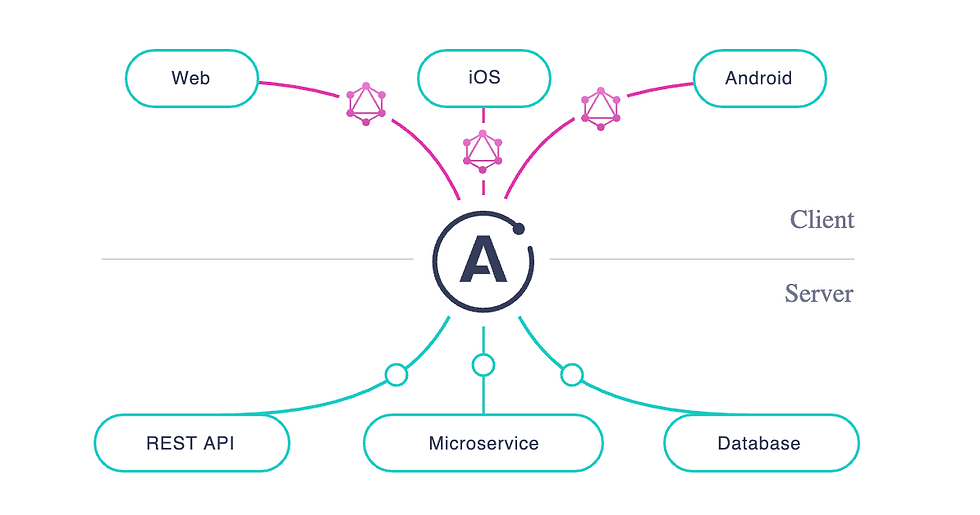
The normal use of Apollo in react is usually through either hooks or through Apollo elements like <Query>. So why would you ever need to access the client directly with something like Thunk?
Progressive Loading
When we large amounts of information, it can be useful to load only key fields on initial load.
const { loading, error, data } = useQuery(GET_USER); useState(() => { if (data) { dispatch(populateUserAdddresses()) } }, [data]);
In this example, populateUserAdddresses, is a function that will be used by our thunk milddleware, but how do we access the Apollo client inside of this thunk?
Well, there's a poorly documented function in the Apollo library that can get us a copy of the context.
import { getApolloContext } from "react-apollo";
getApolloContext gives us the context from the provider creation step in the ApolloProvider bootstrapping (presumably at the root of your app)
In order to use this, we can then call useContext
const apolloClient = useContext(getApolloContext());
Putting it all together
import { getApolloContext } from"react-apollo";
const apolloClient = useContext(getApolloContext());
const { loading, error, data } = useQuery(GET_USER);useState(() => {if (data) {dispatch(populateUserAdddresses(apolloClient)) } }, [data]);
Inside actions.js
export function populateUserAdddresses(client) {
// use the client
return {
type: 'POPULATE_USER_DATA',
payload: extraData
}
}
Now, you can use the "extraData" set in POPULATE_USER_DATA in conjunction with your initial data load to show more fields.
It's a powerful tool to let us query our GraphQL API while we show our most important data first.
Comments